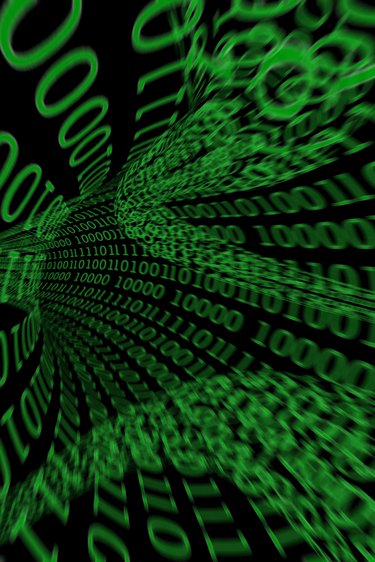
Code::Block is an open source C++ IDE built to meet the complicated development aims of the users. It is developed to be very extensible and configurable. The IDE has the following features: rapid custom build system, support for parallel builds, multi-target projects, user-defined watches, call stack, switch between threads, class browsing and smart indent. MySQL is a popular open source database management system. You can connect to MySQL database in Code::Block and manipulate the data such as retrieve, update, insert and delete.
Step 1
Download Code::Blocks setup file from its official website (see resources.) Double-click the setup file to start the installation. Follow the installation wizard until completion.
Video of the Day
Step 2
Double-click the Code::Blocks icon to enter the development interface. Click "Settings," "Compiler and Debugger" and "Linker Settings." Click "Link Library" tab to open the dialog window. Click "Add" button and input "/usr/lib/libmysqlclient.so."
Step 3
Click "Settings," "Compiler and Debugger" and "Search directories." Select "Compiler" and input "/usr/include/mysql." The development environment with MySQL is properly configured.
Step 4
Include the following files in your application:
#include <mysql.h> #include <MySQLManager.h>
Step 5
Connect to MySQL using the MySQLManager function:
MySQLManager::MySQLManager(string hosts, string userName, string password, string dbName, unsigned int port)
{
IsConnected = false;
this ->setHosts(hosts);
this ->setUserName(username);
this ->setPassword(password);
this ->setDBName(database);
this ->setPort(port); }
Step 6
Conduct SQL queries via function runSQLCommand:
bool MySQLManager::runSQLCommand(string sql)
{
mysql_real_query(&mySQLClient,sql.c_str(),(unsigned int)strlen(sql.c_str())); }
Step 7
Define your Main function using the said functions:
int main(){ MySQLManager sqlres("127.0.0.1","root","search1","HR",3306); sqlres.initConnection(); sqlres.runSQLCommand("select * from employee"); sqlres.destroyConnection(); return 0; }
Video of the Day