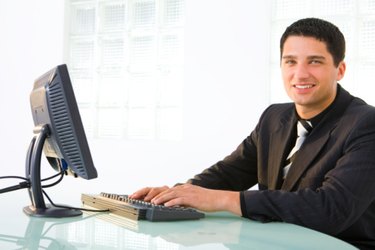
Using null parameters in JavaScript allows programmers to define a variable without assigning a value to it. Null values are used in simple variables like integers and strings, and they are used for objects on a Web page. However, null values become troublesome for calculations or object manipulation. If you have a defined variable in your code, checking for null values prior to executing code limits the number of errors.
Step 1
Declare the variable. Type the following into your JavaScript code:
Video of the Day
var myVal = null;
This allocates memory and defines the variable for use.
Step 2
Evaluate whether the variable is null. In some instances, you may want to execute code if the value is null. Wrap your executable code with this statement:
if (myVal== null) {
}
This statement provides a way to execute code only if myVal is null.
Step 3
Evaluate whether a variable is not null. In other instances, you may want to run code when a value is not null. The "!" operator tells the compiler to run the statements if the result is false. In this statement, JavaScript runs statements if myVal is not null:
if (!name) {
}
Video of the Day