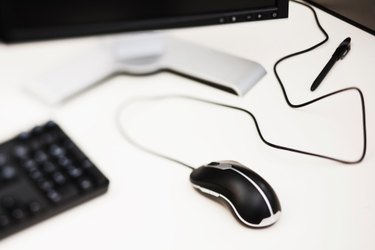
Converting an integer to a string is a common practice when programming. For some application processes, it's necessary to manipulate the format. Java makes converting an integer to a string easy through one of its internal functions.
Step 1
Declare the integer variable. Before using any variable, you need to declare it for the compiler. Here is an example of a defined integer set to the value of 1:
Video of the Day
int myInteger = 1;
Step 2
Declare the string variable. A string variable needs to be defined to save the converted integer. You can't save a string to a declared integer variable, so there needs to be a second variable for the conversion. Here is an example of a declared variable set to a value of an empty string:
String myString = "";
Step 3
Convert the integer to a string. Now the integer can be converted. This line of code converts the myInteger variable and saves it to the myString value:
myString = Integer.toString(myInteger);
Step 4
Print the variable to the console. To verify the conversion, print to the screen. To print in Java, use this code:
System.out.println(myString);
Video of the Day