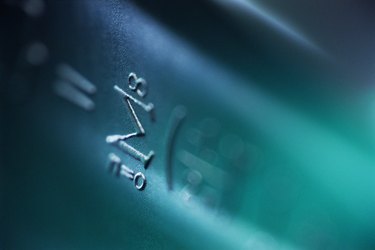
Convolution is a mathematical operation that blends two functions relative to the overlap of one function as it is shifted over another. Although MATLAB contains a pre-built convolution function, it is possible to calculate the discrete convolution integral yourself. The discrete convolution of two functions f and g is defined as the sum over the range 0 to j of f(j) * g(k-j).
Step 1
Define two vectors, f and g, containing the two functions you want to convolve. The lengths of f and g do not have to be equal. The length of result of the convolution, k, will be one less than the sum of the length of f and g:
Video of the Day
m = length(f); n = length(g); k = m + n - 1;
Step 2
Define the range j over which the convolution will occur. The value of j is the range where subscripts of the two functions to be convolved, f(j) and g(k+1-n), are legal. The value of 1 added to k is to account for the fact that MATLAB begins indexing vectors at 1 rather than 0:
j = max(1,k+1-n):min(k,m)
Step 3
Preallocate space for the result of the convolution:
my_result = zeros(k);
Step 4
Write a for loop to iterate through values of k:
for result_index = 1:k
Step 5
Calculate the convolution for all values of j:
my_result(k) = sum(f(j) .* g(k-j+1));
Step 6
Close the for loop with the "end" command.
Video of the Day